第 2 章 Geometries and Aesthetics
Two steps:
Step 1: What geometries do you see?
Step 2: With a given geometry, what aesthetics do you observe?

Geometries?
Aesthetics?
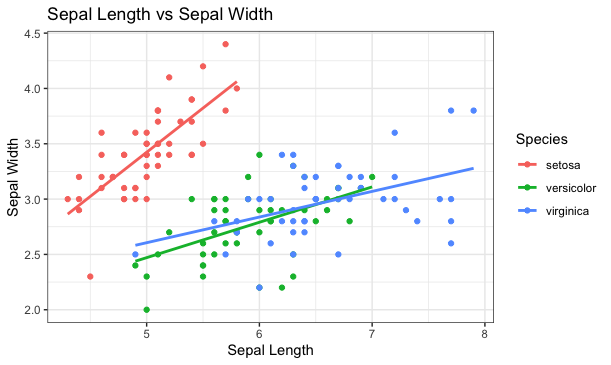
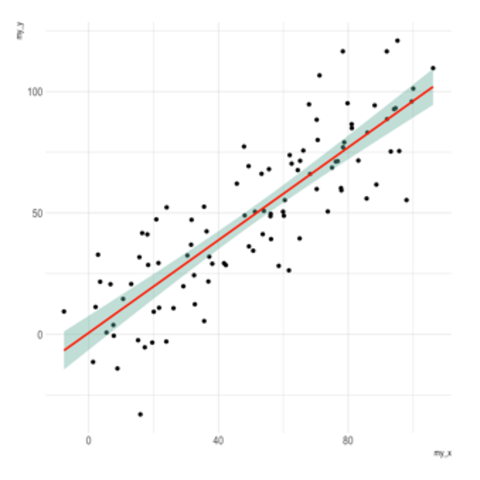

2.1 An Example

Geom:
geom_point
:x, y:varies with observations
color (線框顏色): constant
stroke (線框粗細): constant
fill (內部塗色): constant
size (點的大小): constant
x, y: varies with observations
label: varies with observations
hjust (文字水平錨點):between 0 and 1
vjust (文字垂直錨點): between 0 and 1
# take Colombia, Normway, and Gemany as an example
<- list()
data4plot $point$data <- {
data4plotdata.frame(
x=c(52.5, 56, 58.5),
y=c(48.6, 54.9, 58)
)
}$text$data <- {
data4plotdata.frame(
x=c(52.5, 56, 58.5),
y=c(48.6, 54.9, 58),
label=c("Colombia", "Norway", "Germany")
) }
<- {
myplot ggplot()+
geom_point(
data=data4plot$point$data,
mapping=aes(
x=x, y=y
),fill="blue",
shape=21,
color="black",
stroke=1,
size=1
+
)geom_text(
data=data4plot$text$data,
mapping=aes(
x=x, y=y, label=label
),hjust = 1,
size = 1
)
} myplot
- use
econDV2::ggdash()
to quickly create a shiny app of your ggplot to fine tune your graph.
2.1.1 econDV2::ggdash
<- {
myplot ggplot()+
geom_point(
data=data4plot$point$data,
mapping=aes(
x=x, y=y
),fill="blue", #input$fill_point
shape=21, # default point is a circle without border, you need another type of point
color="black", #input$color_point
stroke=1, #input$stroke_point
size=1 #input$size_point
+
)geom_text(
data=data4plot$text$data,
mapping=aes(
x=x, y=y, label=label
),hjust = 1, #input$hjust_text
size = 1 #input$size_text
)
} myplot
<- {
myplot ggplot() +
geom_point(
data = data4plot$point$data,
mapping = aes(
x = x, y = y
),fill = "#2fc1d3",
shape = 21,
color = "black",
stroke = 1,
size = 12
+
) geom_text(
data = data4plot$text$data,
mapping = aes(
x = x, y = y, label = label
),hjust = 0,
size = 12
)
} myplot
2.1.2 Script saving
If you are happy with your graph, you may want to make it reproducible and easy to use.
- Think of how you want to use it next time.
Turn your script into a function, and save in a R script.
Select the code lines that you want to turn into a function
Code > Extract Function
The result after proper revision:
<- function(data4plot) {
myGoodPlot <- {
myplot ggplot() +
geom_point(
data = data4plot$point$data,
mapping = aes(
x = x, y = y
),fill = "#2fc1d3",
shape = 21,
color = "black",
stroke = 1,
size = 12
+
) geom_text(
data = data4plot$text$data,
mapping = aes(
x = x, y = y, label = label
),hjust = 0,
size = 12
)
}
myplot }
To reuse:
source("support/ggplots.R") # suppose this is where your script file is
<- list()
data4plot $point$data <- {
data4plotdata.frame(
x=c(52.5, 56, 58.5),
y=c(48.6, 54.9, 58)
)
}$text$data <- {
data4plotdata.frame(
x=c(52.5, 56, 58.5),
y=c(48.6, 54.9, 58),
label=c("Colombia", "Norway", "Germany")
)
}
myGoodPlot(data4plot)
- If you replace
data4plot
with the real data, you get the accurate graph that you want.
2.1.3 Graph output
Saving is tricky. You need to find the viewport ratio. (Different computers are different)
Given your graph width and height, you can save your output as:
dir.create("works")
ggsave(
filename = "works/week1.png",
plot=myGoodPlot(data4plot),
width=930,
height=400,
units="mm" # text size is default in mm unit
)
2.1.4 ggdash more
- ggdash can control any input within a programming block
<- {
myplot2 = 21 #input$shape_point
myshape ggplot() +
geom_point(
data = data4plot$point$data,
mapping = aes(
x = x, y = y
),fill = "#2fc1d3",
shape = myshape,
color = "black",
stroke = 1,
size = 12
+
) geom_text(
data = data4plot$text$data,
mapping = aes(
x = x +
0, #input$x_text
y = y, label = label
),hjust = 0,
size = 12
)
} myplot2
2.2 Inheritance
2.2.1 Data
Common data source can be put in
ggplot()
:ALL geoms will use the same data to find aes() mapping,
UNLESS the geom has its own data supplied.
= data4plot$text$data
data
<- {
myplot ggplot(data = data,
+
) geom_point(
mapping = aes(
x = x, y = y
),fill = "#2fc1d3",
shape = 21,
color = "black",
stroke = 1,
size = 12
+
) geom_text(
# data = data4plot$text$data,
mapping = aes(
x = x, y = y, label = label
),hjust = 0,
size = 12
) }
2.2.2 Aesthetics
<- {
myplot ggplot(
data = data,
mapping = aes(x=x, y=y)
+
) geom_point(
fill = "#2fc1d3",
shape = 21,
color = "black",
stroke = 1,
size = 12
+
) geom_text(
mapping = aes(
label = label
),hjust = 0,
size = 12
)
} myplot
<- function(data) {
plot_hightlightCountries <- {
myplot ggplot(data = data,
+
) geom_point(
mapping = aes(
x = x, y = y
),fill = "#2fc1d3",
shape = 21,
color = "black",
stroke = 1,
size = 12
+
) geom_text(
# data = data4plot$text$data,
mapping = aes(
x = x, y = y, label = label
),hjust = 0,
size = 12
)
}
myplot }
2.2.3 Stop data inheritance
- When a geom has its own data supplied, data inheritance stops.
<-
nonhighlightedCountries data.frame(
x=c(48, 52, 53),
y=c(48, 53, 54)
)<- data4plot$text$data
highlightedCountries
<-
plot_allCountries
{plot_hightlightCountries(highlightedCountries) +
geom_point(
data = nonhighlightedCountries,
aes(
x=x,
y=y
),size = 12,
color= "#a4dce6"
)
}
plot_allCountries
<- function(highlightedCountries, nonhighlightedCountries) {
plot_allCountries plot_hightlightCountries(highlightedCountries) +
geom_point(
data = nonhighlightedCountries,
aes(
x=x,
y=y
),size = 12,
color= "#a4dce6"
) }
2.2.4 Finish panel
<- {
plot_obsessionWithBorders plot_allCountries(
hightlightedCountries = highlightedCountries,
unhighlightedCountries = unhighlightedCountries
+
)geom_abline(
aes(
slope=1,
intercept=0
),color = "#549ab6",
linetype = 2,
size=2
)
} plot_obsessionWithBorders
+
plot_obsessionWithBorders geom_abline(
aes(
slope=1,
intercept=0
),color = "#549ab6",
linetype = 2,
size=2
)
<- function(plot_obsessionWithBorders) {
hightlightXYequal +
plot_obsessionWithBorders geom_abline(
aes(
slope=1,
intercept=0
),color = "#549ab6",
linetype = 2,
size=2
) }
2.3 Graph export
The easiest way to export the graph as you see on gg$dash()
is to right click the image on the shiny dashboard and choose save image as to export the image.